함수
- 특정 작업을 수행하는 코드블럭
- 실행시 데이터를 제공해야 하거나. 함수를 호출한 코드에 작업한 결과 반환
- 매개변수(parameter,인자) 인수(argument)는 차이점 있음
메서드
- 특정 클래스, 구조체, 열거형 내의 함수
- 함수를 클래스 내에 선언하면 메서드라 부름
함수를 선언하는 방법.
func <함수명>(<매개변수 이름> : <매개변수 타입>...)> -> <반환값 타입 { 코드 }
func sayHello(){ print("hello") } -> Void // Void는 생략가능.
sayHello() // 호출
print(type(of:sayHello)) // () -> ()
// 하나의 문자열과 하나의 정수를 매개변수로 받아서 문자열을 반환
func message(name : String, age : Int) -> String{
return("\(name \(age)
}
C언어와 Swif함수 변경 연습.
// C , C++
int add(int x, int y){
return(x+y)
}
add(10,20) // 호출
// Swift
func add(x : Int, y : Int) -> Int{
return(x+y) // 괄호 생략가능.
}
add(x : 10, y : 20) // 호출
// 항상 함수를 호출할땐 argument labels를 써줘야 에러가 발생하지 않는다.
print(type(of:add)) // (Int, Int) -> Int
내부 매개변수(parameter)이름과 외부 매개변수(argument label)이름
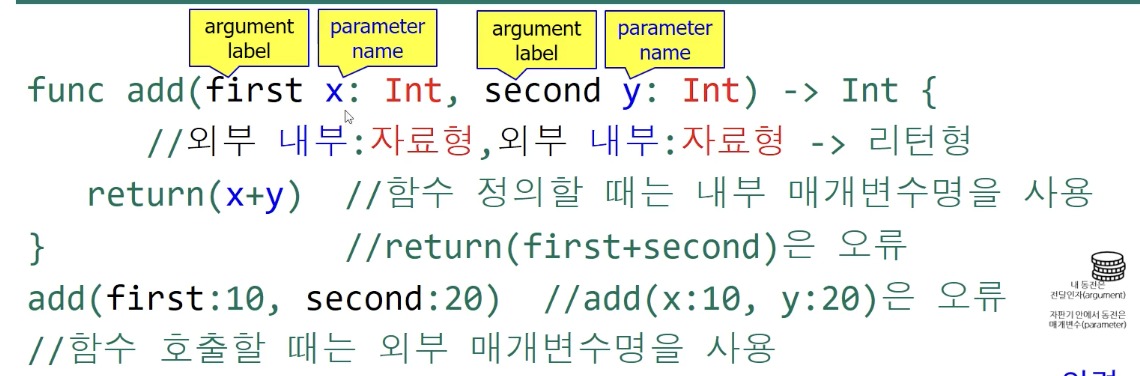
매개변수 1개당 1개만 사용가능!
자료형은 바뀌지않고 똑같다.
Swift함수의 다양한 정의방법
func add(x : Int, y : Int) -> Int{
return(x+y)
}
print(add(x:10,y:20)) // 출력 : 30
print(type(of:add)) // 출력 : (Int, Int) -> Int
// 함수의 이름은 add , 외부매개변수를 생략하면 내부매개변수가 외부까지 겸한다.
// 반환 타입은 Int
func add(first x : Int,second y : Int) -> Int{
return(x+y)
}
print(add(first:10,second:20)) // 출력 : 30
print(type(of:add)) // 출력 : (Int, Int) -> Int
// 외부매개변수는 호출할때사용
// 내부매개변수는 함수내부에서사용
func add(_ x : Int,_ y : Int) -> Int{
return(x+y)
}
print(add(10,20)) // 출력 : 30
print(type(of:add)) // 출력 : (Int, Int) -> Int
// _ 가 나왔다면 외부매개변수를 생략하겠다는 의미
// 결과는 다른언어처럼 잘 나오지만 추천X
func add(_ x : Int, with y : Int) -> Int{
return(x+y)
}
print(add(10,with:20)) // 출력 : 30
print(type(of:add)) // 출력 : (Int, Int) -> Int
// 첫번째 외부매개변수를 생략하는 경우는 많음
// Objective-C언의 호출방식이 이러하다.
// 두번째 매개변수부터는 외부매개변수 사용 -> 제일 많이 사용하는 방식이다.
위 코드의 함수명을 알아보겠습니다.
함수명의 예시 : 함수명(외부매개변수명:외부매개변수명:...)
#function 리터럴 사용시 그 함수의 이름을 결과로 얻을 수 있습니다.
func add1(x : Int, y : Int) -> Int{
print(#function)
return(x+y)
}
let a1 = add1(x:10,y:20) // add1함수를 호출해 리턴값을 a1상수에 할당.
print(a1)
// 출력 : add1(x:y:)
// 출력 : 30
func add2(first x : Int,second y : Int) -> Int{
print(#function)
return(x+y)
}
let a2 = add2(first:10,second:20)
// 출력 : add2(first:second:)
func add3(_ x : Int,_ y : Int) -> Int{
print(#function)
return(x+y)
}
let a3 = add3(10,20)
// 출력 : add3(_:_:)
func add4(_ x : Int, with y : Int) -> Int{
print(#function)
return(x+y)
}
let a4 = add4(10,with:20)
// 출력 : add4(_:with:)
iOS테이블뷰를 설명하는 공식사이트 입니다.
https://developer.apple.com/documentation/uikit/uitableview
iOS 테이블뷰에서 많이 사용하는 메서드를 많이 사용하는 순서대로 나열한 내용입니다.
출처 : perplexity.ai
- cellForRowAt
기능: 각 행에 표시할 셀을 반환
전체 이름: tableView(:cellForRowAt:)
자료형: func tableView( tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCel
// 예시코드
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "CellIdentifier", for: indexPath)
cell.textLabel?.text = "Cell \(indexPath.row)"
return cell
}
- numberOfRowsInSection
기능: 각 섹션에 표시할 행의 개수를 반환
전체 이름: tableView(:numberOfRowsInSection:)
자료형: func tableView( tableView: UITableView, numberOfRowsInSection section: Int) -> Int
// 예시코드
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return dataSource.count
}
- didSelectRowAt
기능: 특정 행이 선택되었을 때 호출되는 메서드
전체 이름: tableView(:didSelectRowAt:)
자료형: func tableView( tableView: UITableView, didSelectRowAt indexPath: IndexPath)
// 예시코드
func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
print("Selected row: \(indexPath.row)")
tableView.deselectRow(at: indexPath, animated: true)
}
- heightForRowAt
기능: 각 행의 높이를 지정
전체 이름: tableView(:heightForRowAt:)
자료형: func tableView( tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat
// 예시코드
func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
return 60.0
}
- numberOfSections
기능: 테이블 뷰의 섹션 수를 반환
전체 이름: numberOfSections(in:)
자료형: func numberOfSections(in tableView: UITableView) -> Int
// 예시코드
func numberOfSections(in tableView: UITableView) -> Int {
return 3
}
참고자료 : 한성현 교수님 수업자료
'iOS프로그래밍 (2학년 2학기)' 카테고리의 다른 글
241017 iOS프로그래밍기초 7주차 (1) | 2024.10.17 |
---|---|
241009 iOS프로그래밍기초 6주차 (1) | 2024.10.14 |
240926 iOS프로그래밍기초 4주차 (3) | 2024.09.26 |
240919 iOS 프로그래밍기초 3주차 (0) | 2024.09.19 |
240912 iOS프로그래밍기초 2주차 (0) | 2024.09.12 |